贪吃蛇游戏是最受欢迎的街机游戏之一。在这款游戏中,玩家的主要目标是在不撞到墙壁或自身的情况下捕捉最多的食物。在学习 Python 或 Pygame 的过程中,可以将制作贪吃蛇游戏作为一项挑战。它是最适合初学者的项目之一,学习制作贪吃蛇游戏是一项既有趣又好玩的事情。
在这篇文章中,我们将使用 Pygame 来制作一个贪吃蛇游戏。Pygame 是一个专为制作视频游戏而设计的开源库。它有内置的图形和声音库,对初学者也很友好,而且可以跨平台使用。
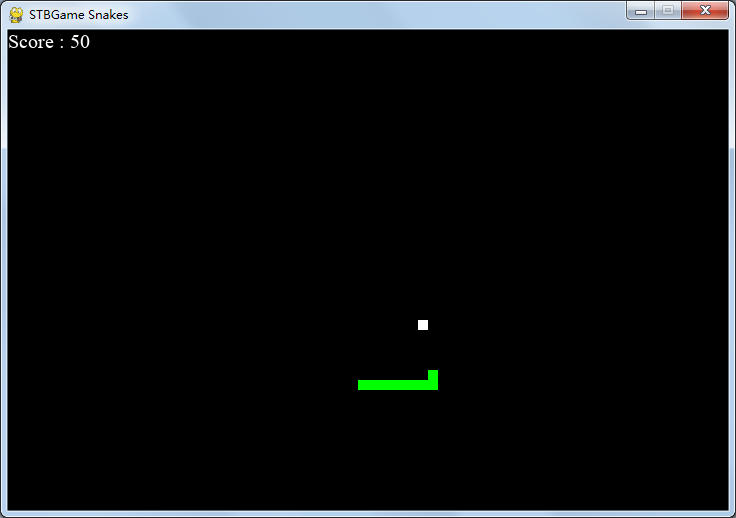
安装:
安装 Pygame时,需要打开命令终端窗口,在窗口中键入以下安装指令:
pip install pygame
如果下载速度太慢,可以在指令中指定使用国内镜像源安装,如清华镜像源:
pip install pygame -i https://pypi.tuna.tsinghua.edu.cn/simple
安装完 Pygame 后,我们就可以开始创建酷酷的贪吃蛇游戏了。
使用 Pygame 一步步创建贪吃蛇游戏:
第一步: 首先,我们要导入必要的程序库。
- 然后,我们将定义游戏窗口的宽度和高度。
- 接着以 RGB 格式定义我们将在游戏中用于显示文本的颜色。
# importing libraries
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
第二步:导入库后,我们需要使用 pygame.init() 方法初始化 Pygame。
- 使用上一步中定义的宽度和高度创建一个游戏窗口。
- 这里pygame.time.Clock() 将用于控制游戏的刷新频率,以改变蛇的速度。
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('STBGame Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
第三步: 初始化蛇的位置和大小。
- 在初始化蛇的位置后,将食物随机设置到游戏窗口内的任意位置。
- 将方向设置为 RIGHT,让用户启动游戏时,小蛇在游戏窗口内向右移动。
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake
# body
snake_body = [ [100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# food position
food_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
food_spawn = True
# setting default snake direction
# towards right
direction = 'RIGHT'
change_to = direction
第四步: 创建一个显示玩家得分的函数。
- 在这个函数中,我们首先创建一个字体对象,通过字体对象可以设置字体的颜色等属性。
- 然后,我们使用 render 创建一个surface显示层,每当用户分数变化时,我们都将更新surface显示层展示新的分数。
- 为得分文本surface显示层对象创建一个矩形object对象(得分文本将在此刷新)。
- 然后,我们调用 blit 函数显示玩家的分数。blit 函数带有两个参数:文本surface显示层和对应的位置。
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the
# text surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
第五步:现在创建一个游戏结束函数,当蛇撞到墙壁或自身后显示得分。
- 在第一行中,我们创建了一个字体object对象,用来显示分数。
- 然后,我们创建得分文本surface表面来呈现分数。
- 然后,我们把文本的位置设置为游戏窗口的中间位置。
- 使用 blit 更新用户得分,并通过 flip() 在游戏窗口中显示分数。
- 我们在调用 quit() 函数关闭游戏窗口前,使用 sleep(2) 等待 2 秒钟。
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render('Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit will draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the
# program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
第六步: 现在,我们将创建游戏的主函数,该函数将执行以下操作:
- 根据玩家在键盘上按下的方向键,设置蛇移动的方向,并设置一个特殊的判断条件,防止蛇向相反方向移动。
- 如果蛇碰到食物,我们将玩家得分递增 10,并在游戏窗口的随机位置创建新的食物。
- 之后,我们检查蛇是否撞到墙壁。如果蛇撞到墙,调用游戏结束函数。
- 检查蛇是否撞到了自己,如果撞到自己,调用游戏结束函数。
- 游戏结束时,使用前面创建的 show_score 函数显示分数。
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two directions
# simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if food and snakes collide then scores will be
# incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == food_position[0] and snake_position[1] == food_position[1]:
score += 10
food_spawn = False
else:
snake_body.pop()
if not food_spawn:
food_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
food_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green, pygame.Rect(
pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
food_position[0], food_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score continuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refresh Rate
fps.tick(snake_speed)
现在,我们已经实现了贪吃蛇游戏的基本功能,游戏的完整代码展示如下:
# importing libraries
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('STBGame Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake body
snake_body = [[100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# food position
food_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
food_spawn = True
# setting default snake direction towards
# right
direction = 'RIGHT'
change_to = direction
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the text
# surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render(
'Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit will draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two
# directions simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if food and snakes collide then scores
# will be incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == food_position[0] and snake_position[1] == food_position[1]:
score += 10
food_spawn = False
else:
snake_body.pop()
if not food_spawn:
food_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
food_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green,
pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
food_position[0], food_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score continuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refresh Rate
fps.tick(snake_speed)
上文中用Python与Pygame实现了贪吃蛇游戏的基本功能,有经验的用户可以下载代码自己运行试玩了,还可以调整游戏中的相关设置改变升级这个游戏。我们也以此为基础,对贪吃蛇游戏进行升级,划分为Snake类,Food类,游戏Setting配置类,更便于新手学习Python编程语言的开发与软件设计的思想。如果需要,请联系站长获取“本游戏”优化版完整代码及《玩游戏学编程》系列中“本项目”对应的详细开发指导教程。
转载请注明:狮驼邦游戏网 » 经典游戏贪吃蛇(Python版)